Guidelines to Master Python Scripting
Welcome to our thorough guide on mastering Python scripting. Recognized for its simplicity and adaptability, Python provides numerous applications for scripting. This article will provide detailed examples and expert advice to help you deeply understand Python scripting.
Decoding Python Scripting
Scripting with Python means creating programs in Python language that automate minor tasks. It’s a proficient method to execute and automate a sequence of commands, which otherwise could be time-consuming.
The Advantages of Selecting Python for Scripting
The easy-to-read syntax, extensive standard library, and wide array of third-party modules make Python an excellent choice for scripting. Its simplicity facilitates swift prototyping while its efficiency helps in handling larger codebases.
Examples of Python Scripting Applications
Let’s examine some practical instances of Python scripting.
File Management through Python Scripting
Python scripting simplifies file management operations such as copying, moving, renaming, and deleting files. Here’s how:
import shutil
# copy file
shutil.copy('source.txt', 'destination.txt')
# move file
shutil.move('source.txt', 'destination_folder')
# rename file
os.rename('old_name.txt', 'new_name.txt')
# delete file
os.remove('file.txt')
Web Scraping using Python Scripting
Python scripting is frequently used for web scraping, i.e., extracting data from websites. Here’s an example using BeautifulSoup:
from bs4 import BeautifulSoup
import requests
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for link in soup.find_all('a'):
print(link.get('href'))
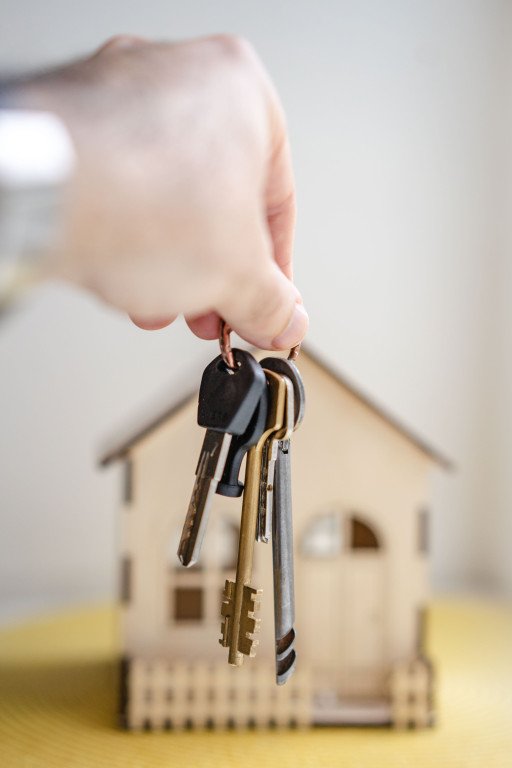
Data Analysis via Python Scripting
Python scripting is potent in managing and analyzing large datasets. Here’s an example using Pandas:
import pandas as pd
# load data
df = pd.read_csv('data.csv')
# summarize data
print(df.describe())
# filter data
filtered_df = df[df['column'] > 100]
# save filtered data
filtered_df.to_csv('filtered_data.csv')
Advanced Applications of Python Scripting
Now, let’s delve into more complex applications of Python scripting.
Python scripting can automate the process of sending emails. This is particularly helpful for sending bulk emails or automated notifications:
import smtplib
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
server.login("your_email", "your_password")
msg = "Hello!"
server.sendmail("your_email", "recipient_email", msg)
server.quit()
Python can be employed to develop web applications. With frameworks like Django or Flask, you can write Python scripts that manage HTTP requests, handle databases, and render HTML pages. Learn more about this from our article on mastering python chess unveiling the tactics and strategies of the game.
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('home.html')
if __name__ == '__main__':
app.run()
Mastering Python scripting equips you with a powerful tool for automating tasks, handling data, and developing applications. With a basic understanding of Python and some practice, you can harness the power of Python scripting to simplify your tasks and solve intricate problems.
Proficiency in Python scripting can pave your way into various fields like data analysis, web development, and system administration. As demonstrated by our examples, the potential uses of Python scripting are expansive and diverse.
We trust this article has given you an extensive understanding of Python scripting and its practical uses. Remember, practice makes perfect when it comes to mastering Python scripting. So, begin with these examples and continue to explore the possibilities offered by Python.
Related Posts
- 7 Steps to Master Hangman Python Programming
- Python Command Line Productivity: 5 Strategies for Developers
- Unveiling the Sophistication of Python Calculator: A Comprehensive Understanding
- A Comprehensive List and Detailed Overview of Python Commands
- Python GUI Development with Tkinter: 5 Essential Tips for Beginners